
Fusce neque. Morbi vestibulum volutpat enim. Curabitur at lacus ac velit ornare lobortis. Morbi ac felis. Curabitur turpis.
Phasellus a est. Praesent adipiscing. Praesent egestas neque eu enim. Maecenas egestas arcu quis ligula mattis placerat. Pellentesque dapibus hendrerit tortor.
Etiam feugiat lorem non metus. Sed a libero. Nam at tortor in tellus interdum sagittis. Suspendisse enim turpis, dictum sed, iaculis a, condimentum nec, nisi. Phasellus gravida semper nisi.
Organiser
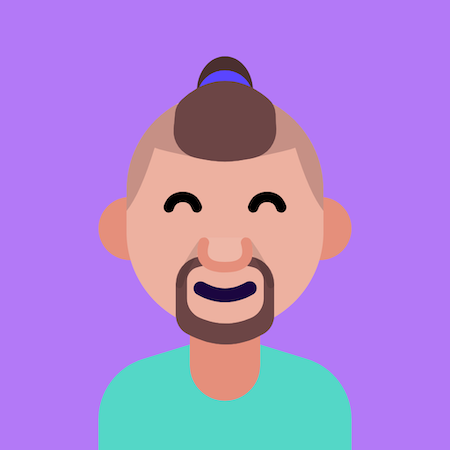
Twitch
const handleAdminSubmitPage =()=>{
if(!window.location.href.includes("admin-activate")) return
const btnSubmitAdmin = document.getElementById('wpforms-submit-6188');
const inputSubmitAdmin = document.getElementById('wpforms-6188-field_1');
const adminParams = new URL(document.location.toString()).searchParams;
const adminSearchParams = new URLSearchParams(adminParams);
const code = adminSearchParams.get('code')
const guildId = adminSearchParams.get('guild_id')
btnSubmitAdmin.addEventListener('click', function (e) {
// e.preventDefault()
// Retrieve the value of the email field
const email = inputSubmitAdmin.value
// Prepare data to send to your localhost server
const data = {
email: email,
guildId,
code
};
console.log({data})
// Configure Fetch API to make a POST request to your localhost server
fetch('https://unicord-6b6b2d12d3a8.herokuapp.com/oauth2/callback', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(function (response) {
console.log({response})
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(function (data) {
// Handle success
console.log('success', data);
})
.catch(function (error) {
// Handle error
console.error('Error sending data to localhost server:', error);
});
});
}
handleAdminSubmitPage()
League of Legends Post-Game Stats
.container {
display: flex;
justify-content: center !important;
align-items: center !important;
flex-direction: column;
font-family: "Lato", Arial, sans-serif;
margin: 10px 50px 10px 50px;
font-size: 1.5rem;
/* Use Lato font as the primary font family */
}
#match-table{
max-width: 90vw !important
}
.hide {
display: none
}
th,
td {
padding: 12px;
text-align: center !important;
vertical-align: middle !important;
}
#stats-table-tbody-blue {
padding-top: 0 !important;
}
.team-row td {
text-align: center;
background-color: #ff7e7e;
/* Fallback color */
background-image: radial-gradient(circle, #ff7e7e, #ff5454);
/* Gradient */
color: white !important;
/* Dark gray font color */
font-size: 30px;
font-weight: 600;
padding: 10px;
padding-top: 0 !important;
margin-top: 0 !important;
margin-bottom: 0 !important;
opacity: 0.8 !important
}
.blue td {
background-color: #7ecbff !important;
padding-top: 0 !important;
background-image: radial-gradient(circle, #7ecbff, #547eff) !important;
color: white !important
}
.team-table {
max-width: 90vw !important;
width: 90vw !important;
}
.focused-player{
color: #C8AA6E;
font-weight: bold;
}
const matchResultsContainer = document.getElementById("match-results")
if(matchResultsContainer) {
matchResultsContainer.classList.add("container");
function fetchMatchData (matchId, puuid, division) {
if (!matchId) return;
fetch("https://unicord-6b6b2d12d3a8.herokuapp.com/match-results?id=" + matchId + "&hashedPuuid=" + puuid + "&division=" + division)
.then(response => response.json())
.then(data => {
const existingTable = document.getElementById('match-table');
if (existingTable) {
existingTable.remove();
}
const matchTable = document.createElement('div');
matchTable.setAttribute('id', 'match-table');
const isMobile = window.innerWidth console.error('Error fetching player data:', error));
}
const createDesktopTable = (matchTable, data) => {
const tableBodyRed = document.createElement('tbody');
const tableBodyBlue = document.createElement('tbody');
// Table Header
const tableHead = document.createElement('thead');
tableHead.innerHTML = `
Role
Player
Uniscore
Level
KDA
Vision Score
Kill Participation
Time Living
Objective Participation
`;
matchTable.appendChild(tableHead);
// Populate Red Team data
const redTeamData = data["100"];
redTeamData.forEach(player => {
const row = document.createElement('tr');
row.innerHTML = `

${player.playerName}
${player.uniscore.toFixed(2)}
${player.level}
${player.kdaString}
${player.visionScore}
${Math.round(player.killParticipation.toFixed(2) * 100)}%
${Math.round(player.livingPercentage.toFixed(2) * 100)}%
${Math.round(player.objectiveParticipation.toFixed(2) * 100)}% `;
tableBodyRed.appendChild(row);
});
// Populate Blue Team data
const blueTeamData = data["200"];
blueTeamData.forEach(player => {
const row = document.createElement('tr');
row.innerHTML = `

${player.playerName}
${player.uniscore.toFixed(2)}
${player.level}
${player.kdaString}
${player.visionScore}
${Math.round(player.killParticipation.toFixed(2) * 100)}%
${Math.round(player.livingPercentage.toFixed(2) * 100)}%
${Math.round(player.objectiveParticipation.toFixed(2) * 100)}% `;
tableBodyBlue.appendChild(row);
});
// Append Red Team header row
const redTeamRow = document.createElement('tr');
redTeamRow.innerHTML = `Red Team `;
redTeamRow.className = "team-row";
matchTable.appendChild(redTeamRow);
matchTable.appendChild(tableBodyRed);
// Append Blue Team header row
const blueTeamRow = document.createElement('tr');
blueTeamRow.innerHTML = `Blue Team `;
blueTeamRow.className = "team-row blue";
matchTable.appendChild(blueTeamRow);
matchTable.appendChild(tableBodyBlue);
}
const createMobileTable = (matchTable, data) => {
const createTeamSection = (teamName, teamData) => {
const teamHeader = document.createElement('h2');
teamHeader.textContent = teamName;
matchTable.appendChild(teamHeader);
teamData.forEach(player => {
const playerBlock = document.createElement('div');
playerBlock.className = "player-block";
playerBlock.innerHTML = `
`;
matchTable.appendChild(playerBlock);
});
}
createTeamSection("Red Team", data["100"]);
createTeamSection("Blue Team", data["200"]);
}
// CSS styles for mobile view
const style = document.createElement('style');
style.innerHTML = `
.player-block {
border: 1px solid #ccc;
padding: 10px;
margin-bottom: 10px;
}
.player-role img {
max-width: 50px;
height: auto;
}
.player-info {
margin-left: 10px;
}
.player-info.focused-player {
background-color: #f0f0f0;
}
@media (min-width: 1000px) {
.player-block {
display: none;
}
}
`;
document.head.appendChild(style);
}
const initSSO = async ()=>{
if(!window.location.href.includes("sso-login-succes")) return
const queryString = window.location.search;
const urlParams = new URLSearchParams(queryString);
const code = urlParams.get('code')
const iss = urlParams.get('iss')
const state = urlParams.get('state')
const session_state = urlParams.get('session_state')
async function agreeFunction() {
const response = await fetch("https://unicord-6b6b2d12d3a8.herokuapp.com/oauth-sso-riot", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify({ code: code, state: state })
});
if (!response.ok) {
throw new Error('Failed to fetch data');
}
const responseData = await response.json();
console.log(responseData);
}
setTimeout(()=> {
console.log(document.querySelector('.termsagree'))
document.querySelector('.termsagree').addEventListener('click', async function(event) {
if (event.target.value === "AGREE") {
event.preventDefault()
const modalWindow = document.getElementById("wp-terms-popup")
modalWindow.style.display = 'none'
await agreeFunction(event);
window.location.href = "https://unicord.gg/sso-login-success/"
}
});
},2000)
}
initSSO()



Player: ${player.playerName}
Uniscore: ${player.uniscore.toFixed(2)}
Level: ${player.level}
KDA: ${player.kdaString}
Vision Score: ${player.visionScore}
Kill Participation: ${Math.round(player.killParticipation.toFixed(2) * 100)}%
Time Living: ${Math.round(player.livingPercentage.toFixed(2) * 100)}%
Objective Participation: ${Math.round(player.objectiveParticipation.toFixed(2) * 100)}%